Use AgilePoint's SendMail API to Send E-mail Notifications
Objective
Provides an example of how to use AgilePoint's SendMail API to send email notifications.
Summary
AgilePoint's SendMail API provides application developers a function to develop custom notification scenarios for applications. For example, it can be used to develop custom e-mail notifications at runtime and/or associate e-mail notifications dynamically to any workflow tasks and/or objects within an application.
There are two SendMail() functions under different classes:
- IWFWorkflowService.SendMail() – This function sends out the e-mail, but does not support attachments. For example, this function is commonly used for an application that is not in-process with AgilePoint Server.
- IWFAPI.SendMail() – This function supports sending out e-mail with attachments, but is only available in system activities. (See Snippets below).
An alternative approach for sending an e-mail with attachments in an AgilePoint Web Application is to use the .NET class, System.Net.Mail.SMTPClient. Please note that for e-mails that are sent out using System.Net.Mail.SMTPClient, AgilePoint Server has no records of these e-mails. If in case you run into e-mail issues for this scenario, the troubleshooting effort should be focused on the custom code in your Web application.
The SMTP Server address is required when using the System.Net.Mail.SMTPClient. The screen captures below provide a sample for how to obtain the SMTP Server address.
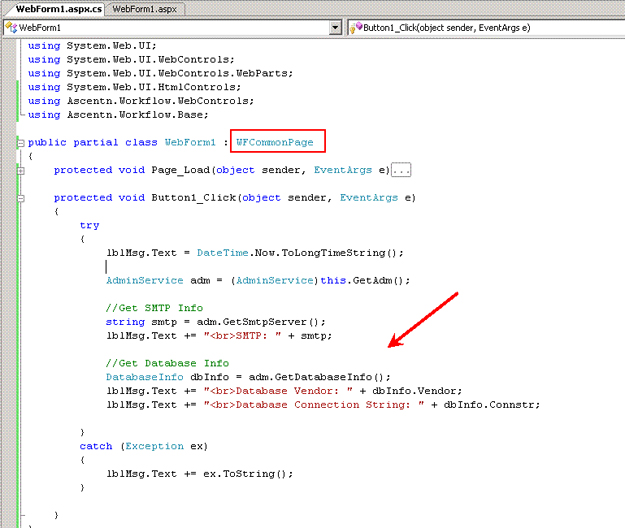
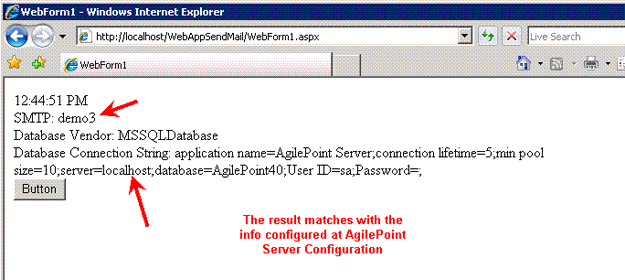
SMTP and database is configured via the AgilePoint Server Configuration.
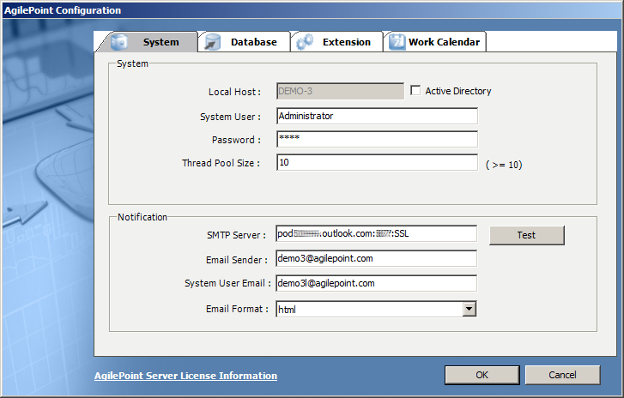
EXAMPLE
The following is a C# code snippet of how the SendMail API is called:
using System;
using System.Collections;
using System.Data;
using System.Xml;
using System.Net;
using System.IO;
using System.Text;
using Ascentn.Workflow.Base;
namespace ManagedCode
{
public class CSharpCodeSnippet
{
// Invoke method is this class's entry point
public void Invoke(
WFProcessInstance pi,
WFAutomaticWorkItem w,
IWFAPI api,
NameValue[] parameters)
{
string emailAddress = WFSystem.SysAdmEMailAddress;
string attachment = WFSystem.HomeDirectory +
"\\License.en-US.RTF";
IWFActivityInstance ai = api.GetActivityInst(w.ActivityInstID);
api.SendMail(
pi,
ai,
emailAddress,
null,
string.Format("Request for Approval - {0}", pi.ProcInstName),
"You are assigned with this task, please
review and approve within two days.",
attachment);
}
}
}